Today I learned that you can run a simple local webserver for static content with only a single line
python -m http.server [<portNo>]
That is simply awesome! It will serve all available files and even list directory contents for you with no problems.
However this basic http.server is very simple and you will quickly run into one of it's biggest drawback which is that it can only handle a single request at a time.
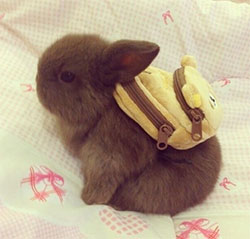
Super adorable, but not very efficient.
Choking on more than one file at a time is not very desirable if you want to be able to make multiple requests within a short time period or if you want more than one user to access the server at a time.
The 10 minute version
Extending the http server code to work for more than a single request at a time is still not very difficult. Below is a fully functioning example of one
#!/bin/env python
import sys, signal
import http.server
import socketserver
# Reading portnumber from command line
if sys.argv[1:]:
port = int(sys.argv[1])
else:
port = 8080
# Note ForkingTCPServer does not work on Windows as the os.fork()
# function is not available on that OS. Instead we must use the
# subprocess server to handle multiple requests
server = socketserver.ThreadingTCPServer(('',port), http.server.SimpleHTTPRequestHandler )
#Ensures that Ctrl-C cleanly kills all spawned threads
server.daemon_threads = True
#Quicker rebinding
server.allow_reuse_address = True
# A custom signal handle to allow us to Ctrl-C out of the process
def signal_handler(signal, frame):
print( 'Exiting http server (Ctrl+C pressed)')
try:
if( server ):
server.server_close()
finally:
sys.exit(0)
# Install the keyboard interrupt handler
signal.signal(signal.SIGINT, signal_handler)
# Now loop forever
try:
while True:
sys.stdout.flush()
server.serve_forever()
except KeyboardInterrupt:
pass
server.server_close()
The code above is based on an example created by Kevin Decherf.
This server can be run directly from the commandline similar to the http.server and with an optional port number as well (assuming the code is stored in the file httpserver.py)
python httpserver.py 8080
This server is actually a pretty good light-weight server for static content. For simple web content such as HTML, JavaScript and stylesheets it works really well. As an in-place testing server I would recommend it.
However it doesn't handle serving large files such as videos and audio very well.
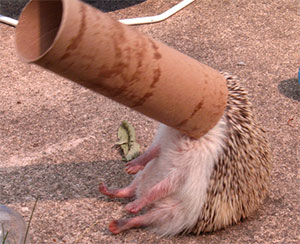
Too big for the inter-connected-network-of-tubes.
Serving large files over HTTP
In the case where you want a simple webserver that handles serving both simple static content and streaming video and audio files I must recommend using the node.js http-server module. It is simply superb.
Assuming you have both node.js and npm installed then it is simply a matter of
npm install http-server -g
And then you can run your webserver directly from the command window like shown below. Just specify which directory you want it to serve content from and the port and you're done
http-server "C:\www\mywebsite" -p 8080
This server handles serving large files (>300MB) quite well, mostly due to the async way that node.js works. But I'm not complaining.
...and it goes really well with that home-made media server website you built!
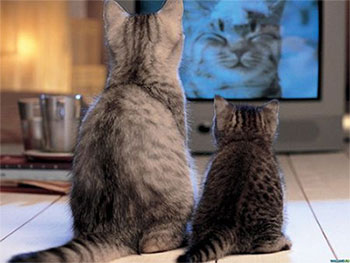
You made a remote as well right?
Developer & Programmer with +15 years professional experience building software.
Seeking WFH, remoting or freelance opportunities.