Recently I wanted to mimic the rather nice non-modal communication through tooltips you get when you click buttons on the GitHub website.
For example when you copy URLs by pressing the copy to clipboard button and then as soon as you click it the tooltip changes to confirm your actions success.
After digging around I found that GitHub uses the jQuery based tipsy library for their tooltips.
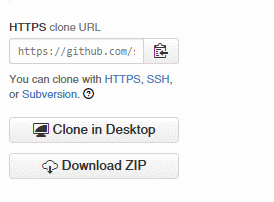
Unfortunately the documentation is incredibly thin and barely covers the most basic use cases.
After an embarrassingly long time I finally landed on a solution that was reasonable for my scenario.
The Challenge
Given my HTML
<button rel='tipsy'
id="copy-button"
data-copied-hint="Copied!"
title="Click to copy url">
<span>TEST</span>
</button>
The main problem was how to instruct the tipsy control to use a different element attribute for the two states (before-copy and then after-copy).
I was unable to instruct the control to read from a different attribute by changing the {title: 'data-copied-hint'}
value on runtime.
My Solution
Luckily the tipsy library accepts functions as the parameters for most of their attributes. So I delegated the creation of the tooltip text to my own function.
var last_clicked_el = null;
function tipsyToolTipText(){
if (this === last_clicked_el) {
last_clicked_el = null;
return this.getAttribute('data-copied-hint');
} else {
return this.getAttribute('original-title');
}
}
// Setup tooltips
$(function () {
$('#copy-button').tipsy({
fade: true,
gravity: 'n',
title: tipsyToolTipText
});
});
// Click hander for button
$("#copy-button").click(function (e) {
last_clicked_el = e.delegateTarget;
$('#copy-button').tipsy("hide");
$('#copy-button').tipsy("show");
});
The messy part is that the page stores the last clicked element as a global variable and it is a very brittle solution and doesn't really scale well.
Not perfect, but hopefully this will help someone along which was previously stuck like me :)
Developer & Programmer with +15 years professional experience building software.
Seeking WFH, remoting or freelance opportunities.