To make it easier to create and enable/disable firewall rules on my router I set out to write a small app that could do all the necessary work for me with a single button press.
My DNSHelper utility has done a good job so far handling the quick switching of DNS entries on my PC network card. But now my DNS provider (Unlocator) requires me to also add a few firewall rules to block a specific set of IP address ranges.
This functionality is out of scope for the DNSHelper so I decided to try to build a sibling app for that.
The Process
I have an Livebox 3 internet router from Orange. This router is used for internet services offered by Orange in France, UK, Spain, Netherlands, Switzerland, Poland and many other countries. Like so many modern routers it offers a web-based management console.
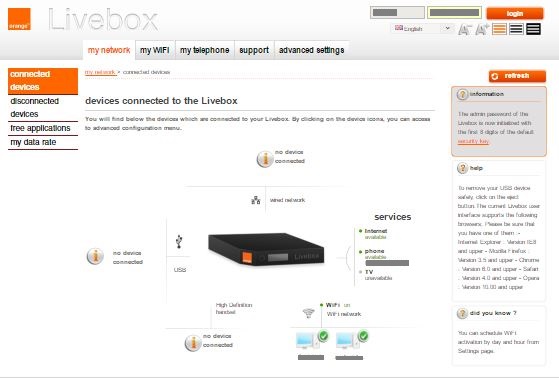
The Livebox 3 Admin Web Console
Using either the Chrome developer console or Fiddler I was able to monitor the HTTP and XMLHttpRequest (XHR) functions used by this web console to pull data out of the router software.
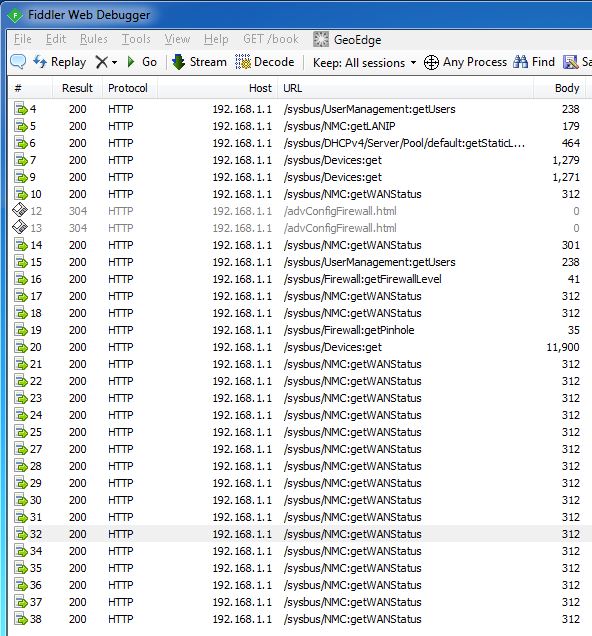
Fiddler trace showing the XHR callback functions used by the Livebox console
From there I built a small C# library that encapsulates these calls and allows you to call them from a .NET project. The library accepts and returns strongly typed objects and makes it quite easy to build your own client front end.
The interesting code can be found in the LiveboxAdapter.cs
class (see the code). This class handles the encapsulation of the XHR calls to the router. The rest of the project is a simple WinForms client example that uses this adapter to manipulate the router.
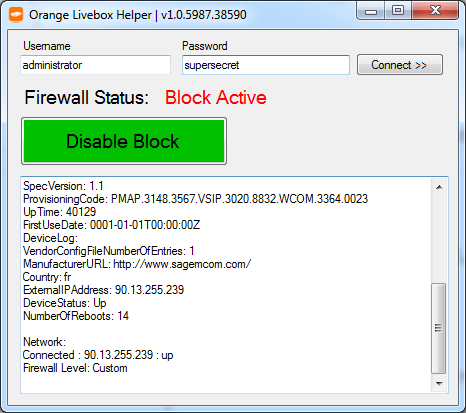
The example project that allows you to switch between custom firewall rules automatically
The code is in its early days and not feature complete yet. As an example, it doesn't yet create the necessary custom firewall rules automatically. However it is complete enough to serve as an example and starting point for other people that might be interested :)
Code Examples
Accessing the router's basic device information
LiveboxAdapter a = new LiveboxAdapter(username, password);
a.LoginAsync().OnSuccess((t, o) =>
{
a.GetDeviceInfo().OnSuccess((t2, o2) =>
{
var res = t2.Result;
// the res object is a typed device info object
}
});
Setting the firewall level to medium
LiveboxAdapter a = new LiveboxAdapter(username, password);
a.LoginAsync().OnSuccess((t, o) =>
{
a.SetFirewallToMedium().OnSuccess((t2, o2) =>
{
var res = t2.Result;
if (!res.Status.GetValueOrDefault())
{
textbox.Text = "Error: " + res.Errors.First().Description;
}
else
{
textbox.Text = "Success: " + res.Status;
}
}, _uiScheduler);
});
Feel free to fork or download the code and play around with it. Just be careful as there is probably a sequence of calls that you can make that could effectively brick your router.
All comments and feedback is greatly appreciated :)
Developer & Programmer with +15 years professional experience building software.
Seeking WFH, remoting or freelance opportunities.